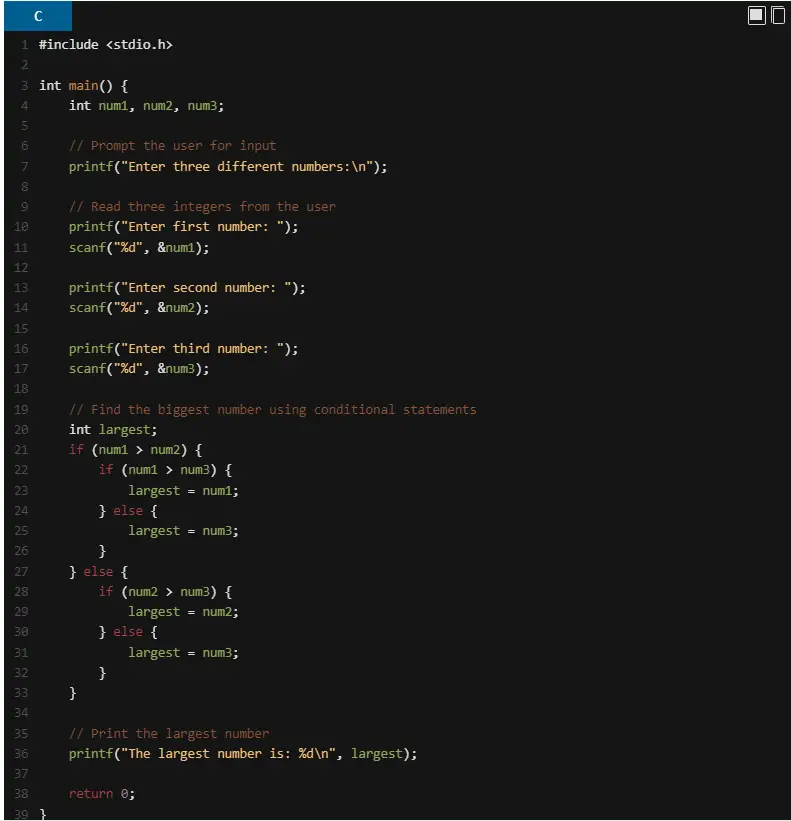
Explanation:
- Header File Inclusion: The
#include <stdio.h>
line includes the standard input-output library necessary for usingprintf
andscanf
functions. - Main Function: The
int main()
is the starting point of the program. - Variable Declaration: Three integer variables
num1
,num2
, andnum3
are declared to store the user inputs. - User Input:
- The program prompts the user to enter three different numbers.
scanf("%d", &num1);
reads the first integer from the user and stores it innum1
.scanf("%d", &num2);
reads the second integer from the user and stores it innum2
.scanf("%d", &num3);
reads the third integer from the user and stores it innum3
.
- Conditional Statements:
- The program uses nested
if
statements to compare the three numbers and determine the largest. - The outer
if
checks ifnum1
is greater thannum2
. - If true, an inner
if
checks ifnum1
is also greater thannum3
to determine ifnum1
is the largest. - If
num1
is not greater thannum3
, thennum3
is the largest. - If the outer
if
is false, another innerif
checks ifnum2
is greater thannum3
to determine ifnum2
is the largest. - If
num2
is not greater thannum3
, thennum3
is the largest.
- The program uses nested
- Print the Largest Number: The
printf
function prints the largest number. - Return Statement: The
return 0;
indicates that the program ended successfully.
When you run this program, it will prompt you to enter three numbers and then display the largest of the three.